JavaScript Intro
This is a very brief introduction to JavaScript. The primary goal is to introduce you to some of the syntax of the JavaScript language. It's ok if you don't understand every part of what's happening.
Basics
In your html documents, you can write JavaScript in a couple of different places.
- Inside the
script
element - In an external JavaScript file
- In the JavaScript Console. This is for testing only as it doesn't actually show on the website.
We can use the console.log() function to log messages to the JavaScript Console. This can be helpful for testing out some code or in debugging. We can log any text we want inside the parenthesis. All we have to do is enclose it in quotation marks.
console.log("Write any text here");
The text will be logged in the JavaScript Console.
Exercise
- Open the JavaScript Intro exercise files.
- Note how the console.log() function is used in
into.html
- Note how the console.log() function is used in
intro.js
- Now use the JavaScript console to type in your own message and view the output.
Variables
I can use the console.log function to log my name like this:
console.log("Dane");
But, that's not terribly useful. What if my name changes? Instead of using a direct input, we often store values in variables so that the values can change without us having to change all of our code.
In JavaScript, you create a variable using the var
keyword.
In the example below, I'm creating a variable named 'name' to be equal to my name.
var name = "Dane";
Now, if I want to use the value of that variable, I can print it to the JavaScript Console. Because it is a variable, I do not use quotation marks around it.
console.log(name);
Text and variables can be combined inside the console.log function with the + symbol to create more complicated messages.
console.log("Hello, " + name);
If I typed the above code directly into the console, it would look like this:
Text and Numbers in JavaScript
When we use characters to create words in JavaScript, they're referred to as strings
. Strings have to be wrapped in quotes (either single or double) to tell them apart from keywords and variable names. Numbers, like variables, don't need quotes.
var voting_age = 18;
You can combine text, numbers, and variables to create more complicated variables.
var message = "In the US you must be " + voting_age + " to vote.";
console.log(message);
Check the console to see the result. Notice how we end each statement with a semicolon.
The value of a variable to can be easily changed. We only use the var keyword the first time we define a variable name.
var fav_color = "blue";
console.log(fav_color);
fav_color = "green";
console.log(fav_color);
Exercise
Use the JavaScript Console to complete the following activities
- Create a variable to store your favorite drink.
- Log a to the console using console.log(). Be sure to use text and the favorite drink variable you created
- Change the value of the favorite drink variable
- Repeat step 2
Functions
We can create functions that contain reusable bits of code that we can then call whenever we want by simply using the function name, followed by parenthesis.
function go() {
console.log("Go bear, Go!");
}
go();
go();
go();
The output in the console should look something like this:
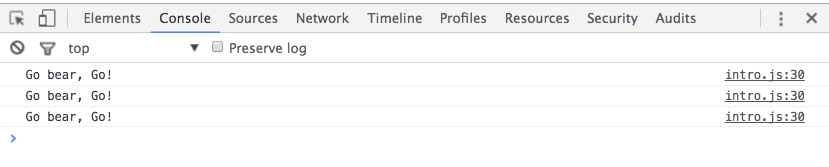
Functions can also take arguments to change or modify. Here's the same function that takes an argument and uses that to modify what it prints to the console. We'll call it three times using different values as the argument.
function go(name) {
console.log("Go bear, Go!");
}
var myName = "Dane";
go(myName);
go("frog");
go("Patriots");
The output in the console should look something like this:

Exercise
Write a function that prints out a welcome to any name give as an argument. The function should take a name as an argument and print a message using console.log. Write the function in intro.js
and then call it 3 times using different argument values. View the results in the JavaScript Console.
Objects
Most of the time in JavaScript we'll be working with some kind of object.
An object can be any type of thing. Take for example, a puppy. Here we'll create a new objected called puppy.
var puppy = new Object();
Properties
Objects can have properties. Our puppy
object could have properties, like names and dog breed. Here, we're setting the properties name
and breed
to string values.
puppy.name = "Fido";
puppy.breed = "cute";
We can access those set properties by using the dot notation.
console.log(puppy.name);
console.log(puppy.breed);
We could save the values of the properties to variables.
var myDogName = puppy.name;
var myDogBreed = puppy.breed;
console.log("My dog's name is " + myDogName + " and it is a " + myDogBreed + ".");
And we can change the value of the properties.
puppy.name = "Petey";
puppy.breed = "cuter";
console.log("My dog's name is " + puppy.name + " and it is a " + puppy.breed + ".");
Exercise
TYPE (don't copy and paste) the above examples into intro.js
. View the console to check the results.
Methods
Objects can also have methods. Methods are something an object does. Methods work a lot like functions.
Let's say our puppy speaks. We'll create a new method called speak, that takes an argument and prints it to the console.
puppy.speak = function(txt) {
console.log(txt);
}
Now we can call the method with different values.
puppy.speak("woof");
puppy.speak("grrr");
Exercise
- Create a new function that takes one argument that will be a number.
- Inside the function, multiply the argument by 2 and save the result to a variable. The multiplication symbol is
*
. Remember, numbers don't need to be enclosed in strings. - Use the
console.log
function to print the value of your variable to the console. - Call the function three times with different numbers.