JavaScript Intro
This is a very brief introduction to JavaScript. The primary goal is to introduce you to some of the syntax of the JavaScript language. It's ok if you don't understand every part of what's happening.
Basics
In most programming languages, we use variables to keep track of things. Below I'm setting a variable to be equal to my name.
var name = "Dane";
Now, if I want to use the value of that variable, I can print it to the web developer console.
console.log("Hello" + name);
The console can be accessed by opening the web developer tools in a browser. In Chrome, click the Console tab in the developer tools to open the console window.
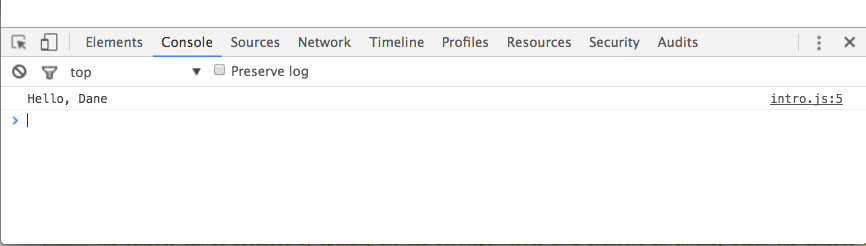
When we use characters to create words in JavaScript, they're referred to as strings. Strings have to be wrapped in quotes (either single or double) to tell them apart from keywords and variable names. Numbers don't need quotes.
var voting_age = 18;
You can add strings and variables together using the plus symbol.
var message = "In the US you must be " + voting_age + " to vote.";
console.log(message);
Check the console to see the result. Notice how we end each statement with a semicolon.
The value of a variable to can be easily changed.
var fav_color = "blue";
console.log(fav_color);
fav_color = "green";
console.log(fav_color);
Functions
We can create functions that contain reusable bits of code that we can then call whenever we want by simply using the function name, followed by parenthesis.
function go() {
console.log("Go bear, Go!");
}
go();
go();
go();
The output in the console should look something like this:
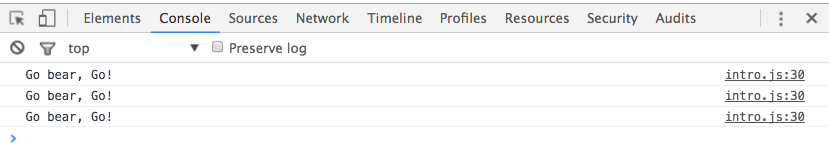
Functions can also take arguments to change or modify. Here's the same function that takes an argument and uses that to modify what it prints to the console. We'll call it three times using different values as the argument.
function go(name) {
console.log("Go bear, Go!");
}
var myName = "Dane";
go(myName);
go("frog");
go("Patriots");
The output in the console should look something like this:

Objects
Most of the time in JavaScript we'll be working with some kind of object.
An object can be any type of thing. Take for example, a puppy. Here we'll create a new objected called puppy.
var puppy = new Object();
Properties
Objects can have properties. Our puppies object could have properties, like names and dog breed. Here, we're setting the attributes name
and breed
to string values.
puppy.name = "Fido";
puppy.breed = "cute";
We can access those set properties by using the dot notation.
console.log(puppy.name);
console.log(puppy.breed);
We could save the values of the properties to variables.
var myDogName = puppy.name;
var myDogBreed = puppy.breed;
console.log("My dog's name is " + myDogName + " and it is a " + myDogBreed + ".");
And we can change the value of the properties.
puppy.name = "Petey";
puppy.breed = "cuter";
console.log("My dog's name is " + puppy.name + " and it is a " + puppy.breed + ".");
Methods
Objects can also have methods. Methods are something an object does. Methods work a lot like functions.
Let's say our puppy speaks. We'll create a new method called speak, that takes an argument and prints it to the console.
puppy.speak = function(txt) {
console.log(txt);
}
Now we can call the method with different values.
puppy.speak("woof");
puppy.speak("grrr");
Exercise 1
- Create a new variable that contains your favorite animal.
- Use the
console.log
function to print the text "My favoriate animal is:" to the console. - Use the
console.log
function to print the value of your variable to the console.
Exercise 2
- Create a new function that takes one argument that will be a number.
- Inside the function, multiply the argument by 2 and save the result to a variable. The multiplication symbol is
*
. Remember, numbers don't need to be enclosed in strings. - Use the
console.log
function to print the value of your variable to the console. - Call the function three times with different numbers.